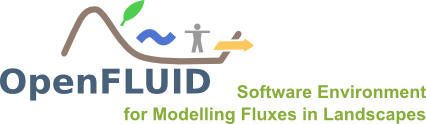
Go to the documentation of this file.
41 #ifndef __THREADEDLOOPMACROS_HPP__
42 #define __THREADEDLOOPMACROS_HPP__
46 #if QT_VERSION >= QT_VERSION_CHECK(5,0,0)
47 #include <QtConcurrent>
50 #include <QtConcurrentRun>
51 #include <QFutureSynchronizer>
60 #define _THREADSYNCID(_id) _M_##_id##_Sync
63 #define _APPLY_UNITS_ORDERED_LOOP_THREADED_WITHID(id,unitclass,funcptr,...) \
64 openfluid::core::UnitsList_t* _UNITSLISTID(id) = mp_CoreData->getUnits(unitclass)->getList(); \
65 if (_UNITSLISTID(id) != NULL) \
67 openfluid::core::UnitsList_t::iterator _UNITSLISTITERID(id) = _UNITSLISTID(id)->begin(); \
68 if (_UNITSLISTITERID(id) != _UNITSLISTID(id)->end()) \
70 openfluid::core::PcsOrd_t _PCSORDID(id) = _UNITSLISTITERID(id)->getProcessOrder(); \
71 while (_UNITSLISTITERID(id) != _UNITSLISTID(id)->end()) \
73 QFutureSynchronizer<void> _THREADSYNCID(id); \
74 while (_UNITSLISTITERID(id) != _UNITSLISTID(id)->end() && _UNITSLISTITERID(id)->getProcessOrder() == _PCSORDID(id)) \
78 _THREADSYNCID(id).addFuture(QtConcurrent::run(boost::bind(&funcptr,this,&(*_UNITSLISTITERID(id)),## __VA_ARGS__)));\
79 if (_THREADSYNCID(id).futures().size() == OPENFLUID_GetSimulatorMaxThreads())\
81 _THREADSYNCID(id).waitForFinished(); \
82 _THREADSYNCID(id).clearFutures(); \
85 catch(QtConcurrent::UnhandledException& E) \
87 throw openfluid::base::FrameworkException("Unknown exception thrown from compute thread"); \
89 ++_UNITSLISTITERID(id); \
91 _THREADSYNCID(id).waitForFinished(); \
92 _THREADSYNCID(id).clearFutures(); \
93 if (_UNITSLISTITERID(id) != _UNITSLISTID(id)->end()) _PCSORDID(id) = _UNITSLISTITERID(id)->getProcessOrder(); \
104 #define APPLY_UNITS_ORDERED_LOOP_THREADED(unitclass,funcptr,...) \
105 _APPLY_UNITS_ORDERED_LOOP_THREADED_WITHID(__LINE__,unitclass,funcptr,## __VA_ARGS__)
109 #define _APPLY_ALLUNITS_ORDERED_LOOP_THREADED_WITHID(id,funcptr,...) \
110 openfluid::core::UnitsPtrList_t* _UNITSPTRLISTID(id) = mp_CoreData->getUnitsGlobally(); \
111 if (_UNITSPTRLISTID(id) != NULL) \
113 openfluid::core::UnitsPtrList_t::iterator _UNITSPTRLISTITERID(id) = _UNITSPTRLISTID(id)->begin(); \
114 if (_UNITSPTRLISTITERID(id) != _UNITSPTRLISTID(id)->end()) \
116 openfluid::core::PcsOrd_t _PCSORDID(id) = (*_UNITSPTRLISTITERID(id))->getProcessOrder(); \
117 while (_UNITSPTRLISTITERID(id) != _UNITSPTRLISTID(id)->end()) \
119 QFutureSynchronizer<void> _THREADSYNCID(id); \
120 while (_UNITSPTRLISTITERID(id) != _UNITSPTRLISTID(id)->end() && (*_UNITSPTRLISTITERID(id))->getProcessOrder() == _PCSORDID(id)) \
124 _THREADSYNCID(id).addFuture(QtConcurrent::run(boost::bind(&funcptr,this,(*_UNITSPTRLISTITERID(id)),## __VA_ARGS__)));\
125 if (_THREADSYNCID(id).futures().size() == OPENFLUID_GetSimulatorMaxThreads())\
127 _THREADSYNCID(id).waitForFinished(); \
128 _THREADSYNCID(id).clearFutures(); \
131 catch(QtConcurrent::UnhandledException& E) \
133 throw openfluid::base::FrameworkException("Unknown exception thrown from compute thread"); \
135 ++_UNITSPTRLISTITERID(id); \
137 _THREADSYNCID(id).waitForFinished(); \
138 _THREADSYNCID(id).clearFutures(); \
139 if (_UNITSPTRLISTITERID(id) != _UNITSPTRLISTID(id)->end()) _PCSORDID(id) = (*_UNITSPTRLISTITERID(id))->getProcessOrder(); \
149 #define APPLY_ALLUNITS_ORDERED_LOOP_THREADED(funcptr,...) \
150 _APPLY_ALLUNITS_ORDERED_LOOP_THREADED_WITHID(__LINE__,funcptr,## __VA_ARGS__)