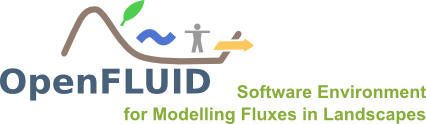
Go to the documentation of this file.
51 #include <openfluid/config.hpp>
53 #define OFDBG_ENABLED 1
54 #define OFDBG_OUTSTREAM std::cout
58 #define OFDBG_ENABLED 0
59 #define OFDBG_OUTSTREAM
70 #define OFDBG_LOCATE { OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " File " << __FILE__ << ", Line " << __LINE__ << std::endl; }
74 #define OFDBG_LOCATE ;
85 #define OFDBG_BANNER \
87 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << std::endl; \
88 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " OpenFLUID debugging mode is enabled" << std::endl; \
89 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << std::endl; \
105 #define OFDBG_MESSAGE(stream) \
106 { OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " " << stream << std::endl; }
110 #define OFDBG_MESSAGE(stream)
121 #define OFDBG_UNIT(unitptr) \
122 { OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " Unit class " << (unitptr)->getClass() << ", ID " << (unitptr)->getID() << std::endl; }
126 #define OFDBG_UNIT(unitptr)
137 #define OFDBG_UNIT_EXTENDED(unitptr) \
139 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " Unit class " << (unitptr)->getClass() << ", ID " << (unitptr)->getID() << std::endl; \
140 std::vector<openfluid::core::AttributeName_t> _M_DBG_AttrsNames = (unitptr)->getAttributes()->getAttributesNames(); \
141 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " - Attributes: "; \
142 for (unsigned int i=0; i<_M_DBG_AttrsNames.size();i++) OFDBG_OUTSTREAM << _M_DBG_AttrsNames[i] << " , "; \
143 OFDBG_OUTSTREAM << std::endl; \
144 std::vector<openfluid::core::VariableName_t> _M_DBG_VarNames = (unitptr)->getVariables()->getVariablesNames(); \
145 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " - Variables: "; \
146 for (unsigned int i=0; i<_M_DBG_VarNames.size();i++) OFDBG_OUTSTREAM << _M_DBG_VarNames[i] << " , "; \
147 OFDBG_OUTSTREAM << std::endl; \
152 #define OFDBG_UNIT_EXTENDED(unitptr)
163 #define OFDBG_EVENT(eventptr) \
165 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " Event at " << (eventptr)->getDateTime().getAsISOString() << std::endl; \
166 openfluid::core::Event::EventInfosMap_t::iterator _M_DBG_EvInfoiter; \
167 for (_M_DBG_EvInfoiter = (eventptr)->getInfos().begin();_M_DBG_EvInfoiter != (eventptr)->getInfos().end();++_M_DBG_EvInfoiter) \
169 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " - " << (*_M_DBG_EvInfoiter).first << " = " << (*_M_DBG_EvInfoiter).second.get() << std::endl; \
175 #define OFDBG_EVENT(eventptr)
186 #define OFDBG_EVENTCOLLECTION(eventcoll) \
188 OFDBG_OUTSTREAM << openfluid::config::DEBUG_PREFIX << " Event collection size : " << eventcoll.getEventsList()->size() << std::endl; \
189 openfluid::core::EventsList_t::iterator _M_DBG_EvListiter; \
190 for (_M_DBG_EvListiter=(EvColl.getEventsList())->begin(); _M_DBG_EvListiter != (EvColl.getEventsList())->end(); _M_DBG_EvListiter++) \
192 OFDBG_EVENT(&(*_M_DBG_EvListiter)); \
198 #define OFDBG_EVENTCOLLECTION(eventcoll)