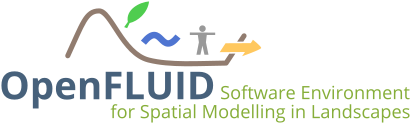 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
39 #ifndef __OPENFLUID_BASE_ENVIRONMENT_HPP__
40 #define __OPENFLUID_BASE_ENVIRONMENT_HPP__
60 static bool m_Initialized;
134 static std::string getWareFullPath(
const std::vector<std::string>& Dirs,
const std::string& Filename);
169 return m_IdealThreadCount;
178 return int(m_IdealThreadCount/2);
205 return m_VersionFull;
214 return m_VersionMajorMinor;
234 return m_InstallPrefix;
243 return m_UserHomeDir;
252 return m_UserDataDir;
260 static std::string getUserDataFullPath(
const std::string& Path);
268 return m_UserExamplesDir;
277 return m_UserExampleSimulatorsDir;
286 return m_ProvidedExamplesDir;
292 static void prepareUserDataDirectory();
309 return m_TranslationsDir;
318 return m_CommonResourcesDir;
326 static std::string getCommonResourcesFullPath(
const std::string& Path);
333 static std::string getAppResourcesDir(
const std::string& AppName);
342 static std::string getAppResourcesFullPath(
const std::string& AppName,
const std::string& Path);
349 static std::vector<std::string> getSimulatorsDirs();
357 return m_DefaultSimulatorsDirs;
366 return m_ExtraSimulatorsDirs;
374 static void addExtraSimulatorsDirs(
const std::string& Paths);
379 static void resetExtraSimulatorsDirs();
385 static std::string getSimulatorFullPath(
const std::string& Filename);
392 static std::vector<std::string> getObserversDirs();
400 return m_DefaultObserversDirs;
409 return m_ExtraObserversDirs;
417 static void addExtraObserversDirs(
const std::string& Paths);
422 static void resetExtraObserversDirs();
428 static std::string getObserverFullPath(
const std::string& Filename);
435 static std::vector<std::string> getBuilderextsDirs();
443 return m_DefaultBuilderextsDirs;
452 return m_ExtraBuilderextsDirs;
460 static void addExtraBuilderextsDirs(
const std::string& Paths);
465 static void resetExtraBuilderextsDirs();
471 static std::string getBuilderextFullPath(
const std::string& Filename);
475 return m_MarketBagBinSubDir;
480 return m_MarketBagBuilderextsDir;
485 return m_MarketBagDataDir;
490 return m_MarketBagDir;
495 return m_MarketBagObserversDir;
500 return m_MarketBagSimulatorsDir;
505 return m_MarketBagSrcSubDir;
510 return m_MarketBagVersionDir;
static std::vector< std::string > m_DefaultObserversDirs
Definition: Environment.hpp:91
static std::string m_MarketBagDir
Definition: Environment.hpp:105
static std::string m_SystemArch
Definition: Environment.hpp:65
static std::string getVersionMajorMinor()
Definition: Environment.hpp:212
#define OPENFLUID_API
Definition: dllexport.hpp:86
static std::string m_ProvidedExamplesDir
Definition: Environment.hpp:99
static std::vector< std::string > getExtraObserversDirs()
Definition: Environment.hpp:407
static std::string getVersionFull()
Definition: Environment.hpp:203
static std::string m_UserExamplesDir
Definition: Environment.hpp:101
static std::string m_VersionFull
Definition: Environment.hpp:73
static std::string m_Version
Definition: Environment.hpp:71
static std::string getUserExamplesDir()
Definition: Environment.hpp:266
static std::string getMarketBagSimulatorsDir()
Definition: Environment.hpp:498
static std::string m_TranslationsDir
Definition: Environment.hpp:121
static std::string m_HostName
Definition: Environment.hpp:67
static std::string getCommonResourcesDir()
Definition: Environment.hpp:316
static std::string getMarketBagDataDir()
Definition: Environment.hpp:483
static std::string m_MarketBagBuilderextsDir
Definition: Environment.hpp:113
static std::string getMarketBagVersionDir()
Definition: Environment.hpp:508
static std::string m_UserHomeDir
Definition: Environment.hpp:81
static std::string m_MarketBagVersionDir
Definition: Environment.hpp:107
static std::string getHostName()
Definition: Environment.hpp:148
Environment()
Definition: Environment.hpp:129
static std::string getTempDir()
Definition: Environment.hpp:222
static std::string m_MarketBagSimulatorsDir
Definition: Environment.hpp:109
static std::string getMarketBagDir()
Definition: Environment.hpp:488
static std::vector< std::string > getExtraSimulatorsDirs()
Definition: Environment.hpp:364
static std::string m_MarketBagDataDir
Definition: Environment.hpp:115
static std::vector< std::string > getDefaultBuilderextsDirs()
Definition: Environment.hpp:441
static std::string m_MarketBagObserversDir
Definition: Environment.hpp:111
static std::string m_MarketBagSrcSubDir
Definition: Environment.hpp:119
Definition: ApplicationException.hpp:47
static std::string m_UserName
Definition: Environment.hpp:69
static std::string getVersion()
Definition: Environment.hpp:194
static std::string getMarketBagBuilderextsDir()
Definition: Environment.hpp:478
static std::string getTranslationsDir()
Definition: Environment.hpp:307
static std::string getSystemArch()
Definition: Environment.hpp:158
static std::string getInstallPrefix()
Definition: Environment.hpp:232
static std::vector< std::string > getDefaultObserversDirs()
Definition: Environment.hpp:398
static std::vector< std::string > m_DefaultSimulatorsDirs
Definition: Environment.hpp:87
static std::string m_UserExampleSimulatorsDir
Definition: Environment.hpp:103
static std::string getUserDataDir()
Definition: Environment.hpp:250
static std::string getMarketBagSrcSubDir()
Definition: Environment.hpp:503
static int m_IdealThreadCount
Definition: Environment.hpp:127
static std::vector< std::string > getExtraBuilderextsDirs()
Definition: Environment.hpp:450
static std::vector< std::string > m_ExtraBuilderextsDirs
Definition: Environment.hpp:97
static std::string getMarketBagBinSubDir()
Definition: Environment.hpp:473
static std::string m_VersionMajorMinor
Definition: Environment.hpp:75
static std::string getUserExampleSimulatorsDir()
Definition: Environment.hpp:275
static std::vector< std::string > m_ExtraObserversDirs
Definition: Environment.hpp:93
static int getIdealJobsCount()
Definition: Environment.hpp:176
static std::string m_UserDataDir
Definition: Environment.hpp:83
static std::string getProvidedExamplesDir()
Definition: Environment.hpp:284
static std::vector< std::string > getDefaultSimulatorsDirs()
Definition: Environment.hpp:355
static std::string m_InstallPrefix
Definition: Environment.hpp:77
Definition: Environment.hpp:52
static int getIdealThreadCount()
Definition: Environment.hpp:167
static std::string getMarketBagObserversDir()
Definition: Environment.hpp:493
static std::string getUserHomeDir()
Definition: Environment.hpp:241
static std::string m_TempDir
Definition: Environment.hpp:79
static std::string getConfigFile()
Definition: Environment.hpp:298
static std::vector< std::string > m_DefaultBuilderextsDirs
Definition: Environment.hpp:95
static std::string m_MarketBagBinSubDir
Definition: Environment.hpp:117
static std::string m_AppsResourcesDir
Definition: Environment.hpp:125
static std::vector< std::string > m_ExtraSimulatorsDirs
Definition: Environment.hpp:89
static std::string m_CommonResourcesDir
Definition: Environment.hpp:123
static std::string getUserName()
Definition: Environment.hpp:185
static std::string m_ConfigFile
Definition: Environment.hpp:85
Generated by
1.8.17