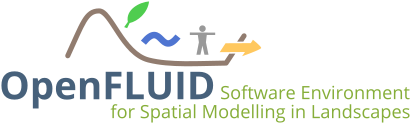 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
40 #ifndef __OPENFLUID_BASE_RUNCONTEXTMANAGER_HPP__
41 #define __OPENFLUID_BASE_RUNCONTEXTMANAGER_HPP__
53 #include <openfluid/ware/TypeDefs.hpp>
68 std::string m_OutputDir;
70 std::string m_InputDir;
72 bool m_IsClearOutputDir;
76 unsigned int m_ValuesBufferSize;
78 unsigned int m_WaresMaxNumThreads;
84 QSettings* mp_ProjectFile;
86 std::string m_ProjectPath;
88 std::string m_ProjectName;
90 std::string m_ProjectDescription;
92 std::string m_ProjectAuthors;
94 std::string m_ProjectCreationDate;
96 std::string m_ProjectLastModDate;
98 bool m_ProjectIncOutputDir;
100 bool m_ProjectIsOpen;
102 static QString m_ProjectFileGroupName;
108 static std::string getNow();
110 void updateWaresEnvironment();
112 static std::string getFilePathFromProjectPath(
const std::string& ProjectPath);
114 static std::string getInputDirFromProjectPath(
const std::string& ProjectPath);
116 static std::string getOuputDirFromProjectPath(
const std::string& ProjectPath);
118 static bool checkProject(
const std::string& ProjectPath);
137 std::string getInputFullPath(
const std::string& Filename)
const;
143 void setInputDir(
const std::string& InputDir);
159 std::string getOutputFullPath(
const std::string& Filename)
const;
165 void setOutputDir(
const std::string& OutputDir);
167 void setDateTimeOutputDir();
175 return m_IsClearOutputDir;
184 m_IsClearOutputDir = Enabled;
193 return m_IsProfiling;
202 m_IsProfiling = Enabled;
211 return m_ValuesBufferSize;
220 m_ValuesBufferSize = Size;
228 { m_ValuesBufferSize = 0; }
236 return (m_ValuesBufferSize > 0);
245 return m_WaresMaxNumThreads;
254 m_WaresMaxNumThreads = Num;
261 void resetWaresMaxNumThreads();
265 return m_ExtraProperties;
270 return m_WaresSharedEnvironment;
277 return m_ProjectPath;
282 return m_ProjectName;
287 m_ProjectName = Name;
292 return m_ProjectDescription;
297 m_ProjectDescription = Description;
302 return m_ProjectAuthors;
307 m_ProjectAuthors = Authors;
312 return m_ProjectCreationDate;
317 m_ProjectCreationDate = CreationDate;
322 m_ProjectCreationDate = getNow();
327 return m_ProjectLastModDate;
332 m_ProjectLastModDate = LastModDate;
337 return m_ProjectIncOutputDir;
342 m_ProjectIncOutputDir = Inc;
345 bool openProject(
const std::string& Path);
347 bool createProject(
const std::string& Path,
const std::string& Name,
348 const std::string& Description,
const std::string& Authors,
353 return m_ProjectIsOpen;
360 static bool isProject(
const std::string& Path);
362 static bool getProjectInfos(
const std::string& Path,
363 std::string& Name, std::string& Description, std::string& Authors,
364 std::string& CreationDate, std::string& LastModDate);
366 void updateProjectOutputDir();
368 QVariant getProjectConfigValue(
const QString& Group,
const QString& Key)
const;
370 void removeProjectConfigValue(
const QString& Group,
const QString& Key);
372 void setProjectConfigValue(
const QString& Group,
const QString& Key,
const QVariant& Value);
std::string getOutputDir() const
Definition: RunContextManager.hpp:149
bool isProfiling() const
Definition: RunContextManager.hpp:191
openfluid::core::MapValue & extraProperties()
Definition: RunContextManager.hpp:263
#define OPENFLUID_API
Definition: dllexport.hpp:86
Definition: MapValue.hpp:92
std::string getProjectLastModDate() const
Definition: RunContextManager.hpp:325
void setWaresMaxNumThreads(unsigned int Num)
Definition: RunContextManager.hpp:252
#define OPENFLUID_SINGLETON_DEFINITION(T)
Definition: SingletonMacros.hpp:55
void setProfiling(bool Enabled)
Definition: RunContextManager.hpp:200
Definition: RunContextManager.hpp:60
void setProjectAuthors(const std::string &Authors)
Definition: RunContextManager.hpp:305
void setProjectCreationDate(const std::string &CreationDate)
Definition: RunContextManager.hpp:315
void setProjectDescription(const std::string &Description)
Definition: RunContextManager.hpp:295
Definition: ApplicationException.hpp:47
void setProjectLastModDate(const std::string &LastModDate)
Definition: RunContextManager.hpp:330
void setProjectIncrementalOutputDir(const bool Inc)
Definition: RunContextManager.hpp:340
void setValuesBufferUserSize(unsigned int Size)
Definition: RunContextManager.hpp:218
const openfluid::core::MapValue & getWaresEnvironment() const
Definition: RunContextManager.hpp:268
bool isValuesBufferUserSize() const
Definition: RunContextManager.hpp:234
std::string getInputDir() const
Definition: RunContextManager.hpp:127
unsigned int getValuesBufferUserSize() const
Definition: RunContextManager.hpp:209
void setProjectName(const std::string &Name)
Definition: RunContextManager.hpp:285
void unsetValuesBufferUserSize()
Definition: RunContextManager.hpp:227
bool isClearOutputDir() const
Definition: RunContextManager.hpp:173
std::string getProjectDescription() const
Definition: RunContextManager.hpp:290
Definition: Environment.hpp:52
void setProjectCreationDateAsNow()
Definition: RunContextManager.hpp:320
std::string getProjectPath() const
Definition: RunContextManager.hpp:275
std::map< WareParamKey_t, WareParamValue_t > WareParams_t
Definition: TypeDefs.hpp:146
std::string getProjectCreationDate() const
Definition: RunContextManager.hpp:310
unsigned int getWaresMaxNumThreads() const
Definition: RunContextManager.hpp:243
bool isProjectIncrementalOutputDir() const
Definition: RunContextManager.hpp:335
std::string getProjectName() const
Definition: RunContextManager.hpp:280
bool isProjectOpen() const
Definition: RunContextManager.hpp:351
void setClearOutputDir(bool Enabled)
Definition: RunContextManager.hpp:182
std::string getProjectAuthors() const
Definition: RunContextManager.hpp:300
Generated by
1.8.17