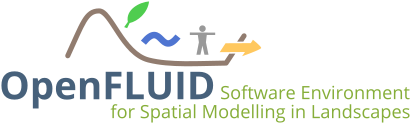 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
40 #ifndef __OPENFLUID_CORE_DATETIME_HPP__
41 #define __OPENFLUID_CORE_DATETIME_HPP__
44 #include <sys/types.h>
49 #include <openfluid/core/TypeDefs.hpp>
114 void updateYMDHMSFromRawTime();
116 void updateRawTimeFromYMDHMS();
139 DateTime(
int Year,
int Month,
int Day,
int Hour,
int Minute,
int Second);
158 bool set(
int Year,
int Month,
int Day,
int Hour,
int Minute,
int Second);
164 void set(
const RawTime_t& SecondsSince0000);
170 bool setFromISOString(
const std::string& DateTimeStr);
176 bool setFromString(
const std::string& DateTimeStr,
const std::string& FormatStr);
185 return (m_TM.tm_year+1900);
194 return (m_TM.tm_mon+1);
244 std::string getAsISOString()
const;
251 std::string getAsString(
const std::string& Format)
const;
258 std::string getDateAsISOString()
const;
264 std::string getTimeAsISOString()
const;
270 void addSeconds(
const RawTime_t& Seconds);
275 void subtractSeconds(
const RawTime_t& Seconds);
295 bool operator==(
const DateTime& Right)
const;
300 bool operator!=(
const DateTime& Right)
const;
305 bool operator>(
const DateTime& Right)
const;
310 bool operator>=(
const DateTime& Right)
const;
315 bool operator<(
const DateTime& Right)
const;
320 bool operator<=(
const DateTime& Right)
const;
354 {
return (3600*N); };
366 {
return (86400*N); };
378 {
return (604800*N); };
383 static bool isLeapYear(
int Year);
388 static int getNumOfDaysInMonth(
int Year,
int Month);
393 static bool isValidDateTime(
int Year,
int Month,
int Day,
int Hour,
int Minute,
int Second);
398 bool isSameDate(
const DateTime& DT)
const;
403 bool isSameTime(
const DateTime& DT)
const;
int getYear() const
Definition: DateTime.hpp:183
#define OPENFLUID_API
Definition: dllexport.hpp:86
static RawTime_t Day()
Definition: DateTime.hpp:359
static RawTime_t Days(int N)
Definition: DateTime.hpp:365
int getDay() const
Definition: DateTime.hpp:201
static RawTime_t Minutes(int N)
Definition: DateTime.hpp:341
int getMonth() const
Definition: DateTime.hpp:192
static RawTime_t Hour()
Definition: DateTime.hpp:347
static RawTime_t Minute()
Definition: DateTime.hpp:335
int getMinute() const
Definition: DateTime.hpp:219
Definition: ApplicationException.hpp:47
int getSecond() const
Definition: DateTime.hpp:228
static RawTime_t Hours(int N)
Definition: DateTime.hpp:353
std::uint64_t RawTime_t
Definition: TypeDefs.hpp:284
int getHour() const
Definition: DateTime.hpp:210
static RawTime_t Weeks(int N)
Definition: DateTime.hpp:377
Class for management of date and time information.
Definition: DateTime.hpp:87
static RawTime_t Week()
Definition: DateTime.hpp:371