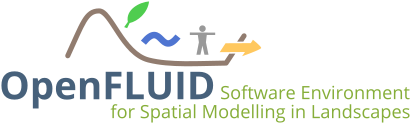 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
40 #ifndef __OPENFLUID_CORE_VECTOR_HPP__
41 #define __OPENFLUID_CORE_VECTOR_HPP__
73 bool allocate(
unsigned long Size);
95 Vector(
unsigned long Size);
100 Vector(
unsigned long Size,
const T& InitValue);
105 Vector(T* Data,
unsigned long Size);
120 virtual ~
Vector() =
default;
151 void setData(T* Data,
unsigned long Size);
156 T getElement(
unsigned long Index)
const;
161 inline T
at(
unsigned long Index)
const
163 return getElement(Index);
169 inline T
get(
unsigned long Index)
const
171 return getElement(Index);
178 void setElement(
unsigned long Index,
const T& Val);
183 inline void set(
unsigned long Index,
const T& Val)
185 setElement(Index,Val);
192 T& operator[](
unsigned long Index);
198 void fill(
const T& Val);
230 return &m_Data[m_Size];
237 inline const_iterator
end()
const
239 return &m_Data[m_Size];
265 if (!allocate(Other.
m_Size))
307 std::fill(m_Data.get(), m_Data.get() + Size, InitValue);
326 std::copy(Data, Data + Size, m_Data.get());
359 m_Size = Other.m_Size;
360 m_Data = std::move(Other.m_Data);
379 m_Data = std::move(std::make_unique<T[]>(Size));
409 std::copy(Data, Data + Size, m_Data.get());
425 return m_Data[Index];
457 return m_Data[Index];
480 std::fill(m_Data.get(), m_Data.get() + m_Size,Val);
#define OPENFLUID_API
Definition: dllexport.hpp:86
const typedef T * const_iterator
Definition: Vector.hpp:64
void fill(const T &Val)
Definition: Vector.hpp:478
void init()
Definition: Vector.hpp:466
T * data() const
Definition: Vector.hpp:143
bool allocate(unsigned long Size)
Definition: Vector.hpp:374
unsigned long m_Size
Definition: Vector.hpp:71
Definition: Vector.hpp:58
Definition: ApplicationException.hpp:47
Vector< T > & operator=(const Vector &Other)
Definition: Vector.hpp:335
unsigned long getSize() const
Definition: Vector.hpp:126
std::unique_ptr< T[]> m_Data
Definition: Vector.hpp:69
T getElement(unsigned long Index) const
Definition: Vector.hpp:418
static void copy(const Vector &Source, Vector &Dest)
Definition: Vector.hpp:500
unsigned long size() const
Definition: Vector.hpp:135
void setData(T *Data, unsigned long Size)
Definition: Vector.hpp:400
const_iterator begin() const
Definition: Vector.hpp:219
T * iterator
Definition: Vector.hpp:62
Definition: FrameworkException.hpp:50
T get(unsigned long Index) const
Definition: Vector.hpp:169
void set(unsigned long Index, const T &Val)
Definition: Vector.hpp:183
void clear()
Definition: Vector.hpp:489
iterator end()
Definition: Vector.hpp:228
T at(unsigned long Index) const
Definition: Vector.hpp:161
iterator begin()
Definition: Vector.hpp:210
void setElement(unsigned long Index, const T &Val)
Definition: Vector.hpp:434
T & operator[](unsigned long Index)
Definition: Vector.hpp:450
const_iterator end() const
Definition: Vector.hpp:237
Vector()
Definition: Vector.hpp:250
Generated by
1.8.17