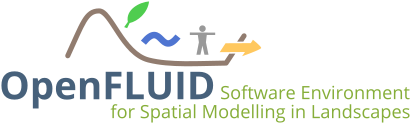 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
40 #ifndef __OPENFLUID_UTILS_FLUIDHUBAPICLIENT_HPP__
41 #define __OPENFLUID_UTILS_FLUIDHUBAPICLIENT_HPP__
49 #include <openfluid/ware/TypeDefs.hpp>
77 typedef std::map<openfluid::ware::WareType,std::set<openfluid::ware::WareID_t>>
WaresListByType_t;
92 QString m_HubAPIVersion;
94 bool m_IsV0ofAPI =
true;
96 QString m_WareCapabilityName =
"wareshub";
98 std::set<QString> m_HubCapabilities;
102 bool isCapable(
const QString& Capacity)
const;
138 return !(m_RESTClient.
getBaseURL().isEmpty());
165 return m_HubAPIVersion;
192 return m_HubCapabilities;
199 std::string getUserUnixname(std::string Email, std::string Password);
205 WaresListByType_t getAllAvailableWares()
const;
214 const QString& Username =
"")
const;
221 QString getNews(
const QString& Lang =
"")
const;
Definition: FluidHubAPIClient.hpp:56
std::set< std::string > ROUsers
Definition: FluidHubAPIClient.hpp:72
Definition: RESTClient.hpp:113
#define OPENFLUID_API
Definition: dllexport.hpp:86
bool isV0ofAPI() const
Definition: FluidHubAPIClient.hpp:145
QString getBaseURL() const
Definition: RESTClient.hpp:152
bool isConnected() const
Definition: FluidHubAPIClient.hpp:136
WareType
Definition: TypeDefs.hpp:60
std::string GitUrl
Definition: FluidHubAPIClient.hpp:66
std::string ShortDescription
Definition: FluidHubAPIClient.hpp:64
Definition: FluidHubAPIClient.hpp:60
QString getHubName() const
Definition: FluidHubAPIClient.hpp:181
Definition: ApplicationException.hpp:47
std::map< openfluid::ware::WareType, std::set< openfluid::ware::WareID_t > > WaresListByType_t
Definition: FluidHubAPIClient.hpp:77
std::set< std::string > RWUsers
Definition: FluidHubAPIClient.hpp:74
QString getHubStatus() const
Definition: FluidHubAPIClient.hpp:172
std::set< QString > getHubCapabilities() const
Definition: FluidHubAPIClient.hpp:190
std::map< std::string, unsigned int > IssuesCounters
Definition: FluidHubAPIClient.hpp:70
~FluidHubAPIClient()
Definition: FluidHubAPIClient.hpp:111
QString getHubAPIVersion() const
Definition: FluidHubAPIClient.hpp:163
std::map< openfluid::ware::WareID_t, WareDetailedDescription > WaresDetailsByID_t
Definition: FluidHubAPIClient.hpp:79
QString getHubURL() const
Definition: FluidHubAPIClient.hpp:154
std::vector< std::string > GitBranches
Definition: FluidHubAPIClient.hpp:68
Definition: RESTClient.hpp:62
Generated by
1.8.17