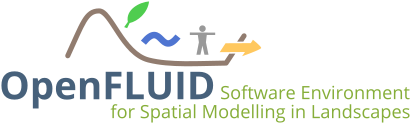 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
40 #ifndef __OPENFLUID_UTILS_RESTCLIENT_HPP__
41 #define __OPENFLUID_UTILS_RESTCLIENT_HPP__
44 #if QT_VERSION >= QT_VERSION_CHECK(5, 6, 0)
45 # define OPENFLUID_REST_URL_REDIRECT 1
47 # define OPENFLUID_REST_URL_REDIRECT 0
71 int m_StatusCode = -1;
73 unsigned int m_NetworkErrorCode = 0;
75 QString m_NetworkErrorString;
84 Reply(
int StatusCode,
unsigned int NetworkErrorCode,
const QString& NetworkErrorString,
const QString& Content):
85 m_StatusCode(StatusCode),
86 m_NetworkErrorCode(NetworkErrorCode),
87 m_NetworkErrorString(NetworkErrorString),
92 {
return m_StatusCode; }
95 {
return m_NetworkErrorCode; }
98 {
return m_NetworkErrorString; }
101 {
return m_Content; }
104 {
return m_NetworkErrorCode == 0 && m_StatusCode >= 200 && m_StatusCode < 300; }
117 QSslSocket::PeerVerifyMode m_CertificateVerifyMode;
125 {
return m_CertificateVerifyMode; }
128 { m_CertificateVerifyMode = Mode; }
136 SSLConfiguration m_SSLConfiguration;
140 Reply performRequest(
const QString& Path,
const QString& Method,
const QString& Data =
"")
const;
150 void setBaseURL(
const QString& URL);
153 {
return m_BaseURL; }
156 { m_SSLConfiguration = Config; }
158 void resetRawHeaders();
160 void removeRawHeader(
const QByteArray Key);
162 bool hasRawHeader(
const QByteArray Key);
164 void addRawHeader(
const QByteArray Key,
const QByteArray Value);
167 {
return m_SSLConfiguration; }
169 Reply getResource(
const QString& Path)
const;
171 Reply postResource(
const QString& Path,
const QString& Data)
const;
173 Reply putResource(
const QString& Path,
const QString& Data)
const;
175 Reply patchResource(
const QString& Path,
const QString& Data)
const;
177 Reply deleteResource(
const QString& Path,
const QString& Data)
const;
Definition: RESTClient.hpp:113
#define OPENFLUID_API
Definition: dllexport.hpp:86
QString getContent() const
Definition: RESTClient.hpp:100
Reply(int StatusCode, unsigned int NetworkErrorCode, const QString &NetworkErrorString, const QString &Content)
Definition: RESTClient.hpp:84
QString getBaseURL() const
Definition: RESTClient.hpp:152
QSslSocket::PeerVerifyMode getCertificateVerifyMode() const
Definition: RESTClient.hpp:124
SSLConfiguration()
Definition: RESTClient.hpp:121
int getNetworkErrorCode() const
Definition: RESTClient.hpp:94
void clear()
Definition: RESTClient.hpp:106
int getStatusCode() const
Definition: RESTClient.hpp:91
bool isOK() const
Definition: RESTClient.hpp:103
QString getNetworkErrorString() const
Definition: RESTClient.hpp:97
Definition: ApplicationException.hpp:47
~RESTClient()
Definition: RESTClient.hpp:147
Definition: RESTClient.hpp:67
void setSSLConfiguration(const SSLConfiguration &Config)
Definition: RESTClient.hpp:155
void setCertificateVerifyMode(QSslSocket::PeerVerifyMode Mode)
Definition: RESTClient.hpp:127
SSLConfiguration getSSLConfiguration() const
Definition: RESTClient.hpp:166
std::list< std::pair< QByteArray, QByteArray > > t_HeadersList
Definition: RESTClient.hpp:60
Definition: RESTClient.hpp:62
Generated by
1.8.17