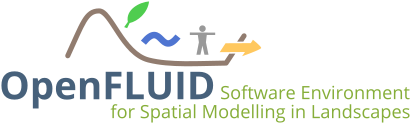 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
39 #ifndef __OPENFLUID_WARE_SIMULATORSIGNATURE_HPP__
40 #define __OPENFLUID_WARE_SIMULATORSIGNATURE_HPP__
43 #include <openfluid/config.hpp>
45 #include <openfluid/core/TypeDefs.hpp>
76 DataName(
""),Description(
""),DataUnit(
"")
80 DataName(DName),Description(DDescription),DataUnit(DUnit)
103 std::string DDescription, std::string DUnit) :
128 std::string DDescription, std::string DUnit);
155 std::vector<SignatureTypedSpatialDataItem>
UsedVars;
179 RequiredParams.clear();
180 ProducedVars.clear();
182 RequiredVars.clear();
184 ProducedAttribute.clear();
185 RequiredAttribute.clear();
186 UsedAttribute.clear();
187 RequiredExtraFiles.clear();
188 UsedExtraFiles.clear();
189 UsedEventsOnUnits.clear();
211 UnitsClass(
""),Description(
"")
215 UnitsClass(UClass),Description(DDescription)
241 UpdatedUnitsGraph.clear();
242 UpdatedUnitsClass.clear();
357 HandledUnitsGraph.
clear();
SignatureUnitsClassItem()
Definition: SimulatorSignature.hpp:210
std::string SimMethod_t
Definition: SimulatorSignature.hpp:53
Definition: SimulatorSignature.hpp:139
std::vector< SignatureSpatialDataItem > RequiredAttribute
Definition: SimulatorSignature.hpp:159
SignatureUnitsGraph()
Definition: SimulatorSignature.hpp:233
std::vector< std::string > RequiredExtraFiles
Definition: SimulatorSignature.hpp:163
std::string DataName
Definition: SimulatorSignature.hpp:71
SignatureSpatialDataItem()
Definition: SimulatorSignature.hpp:98
std::vector< SignatureDataItem > RequiredParams
Definition: SimulatorSignature.hpp:147
std::string SimDomain_t
Definition: SimulatorSignature.hpp:57
SignatureTimeScheduling TimeScheduling
Definition: SimulatorSignature.hpp:341
void setAsFixed(openfluid::core::Duration_t Val)
Definition: SimulatorSignature.hpp:283
#define OPENFLUID_API
Definition: dllexport.hpp:86
std::vector< SignatureTypedSpatialDataItem > UpdatedVars
Definition: SimulatorSignature.hpp:151
SimDomain_t Domain
Definition: SimulatorSignature.hpp:316
std::string UpdatedUnitsGraph
Definition: SimulatorSignature.hpp:228
Definition: WareSignature.hpp:52
std::vector< SignatureSpatialDataItem > UsedAttribute
Definition: SimulatorSignature.hpp:161
void setAsUndefined()
Definition: SimulatorSignature.hpp:269
SignatureHandledData()
Definition: SimulatorSignature.hpp:170
std::string Description
Definition: SimulatorSignature.hpp:72
std::vector< SignatureTypedSpatialDataItem > UsedVars
Definition: SimulatorSignature.hpp:155
Type
Definition: Value.hpp:66
std::vector< SignatureSpatialDataItem > ProducedAttribute
Definition: SimulatorSignature.hpp:157
SimProcess_t Process
Definition: SimulatorSignature.hpp:321
openfluid::core::UnitsClass_t UnitsClass
Definition: SimulatorSignature.hpp:206
SchedulingType Type
Definition: SimulatorSignature.hpp:257
Definition: SimulatorSignature.hpp:224
openfluid::core::Duration_t Min
Definition: SimulatorSignature.hpp:259
SignatureDataItem()
Definition: SimulatorSignature.hpp:75
@ UNDEFINED
Definition: SimulatorSignature.hpp:255
openfluid::core::Duration_t Max
Definition: SimulatorSignature.hpp:261
SignatureTypedSpatialDataItem()
Definition: SimulatorSignature.hpp:123
void clear()
Definition: SimulatorSignature.hpp:350
Definition: SimulatorSignature.hpp:67
SimulatorSignature()
Definition: SimulatorSignature.hpp:344
Definition: SimulatorSignature.hpp:308
SignatureTimeScheduling()
Definition: SimulatorSignature.hpp:264
Definition: SimulatorSignature.hpp:202
std::string Description
Definition: SimulatorSignature.hpp:208
SignatureSpatialDataItem(std::string DName, openfluid::core::UnitsClass_t UClass, std::string DDescription, std::string DUnit)
Definition: SimulatorSignature.hpp:102
Definition: SimulatorSignature.hpp:116
void setAsDefaultDeltaT()
Definition: SimulatorSignature.hpp:276
std::string SimProcess_t
Definition: SimulatorSignature.hpp:55
Definition: SimulatorSignature.hpp:251
Definition: ApplicationException.hpp:47
SchedulingType
Definition: SimulatorSignature.hpp:255
SignatureDataItem(std::string DName, std::string DDescription, std::string DUnit)
Definition: SimulatorSignature.hpp:79
openfluid::core::UnitsClass_t UnitsClass
Definition: SimulatorSignature.hpp:96
SignatureUnitsClassItem(openfluid::core::UnitsClass_t UClass, std::string DDescription)
Definition: SimulatorSignature.hpp:214
std::string DataUnit
Definition: SimulatorSignature.hpp:73
SignatureUnitsGraph HandledUnitsGraph
Definition: SimulatorSignature.hpp:336
std::string UnitsClass_t
Definition: TypeDefs.hpp:98
std::vector< openfluid::core::UnitsClass_t > UsedEventsOnUnits
Definition: SimulatorSignature.hpp:167
std::uint64_t Duration_t
Definition: TypeDefs.hpp:312
void setAsRange(openfluid::core::Duration_t MinVal, openfluid::core::Duration_t MaxVal)
Definition: SimulatorSignature.hpp:290
std::vector< std::string > UsedExtraFiles
Definition: SimulatorSignature.hpp:165
void clear()
Definition: WareSignature.hpp:99
SimMethod_t Method
Definition: SimulatorSignature.hpp:326
void clear()
Definition: SimulatorSignature.hpp:239
SignatureHandledData HandledData
Definition: SimulatorSignature.hpp:331
openfluid::core::Value::Type DataType
Definition: SimulatorSignature.hpp:121
Definition: SimulatorSignature.hpp:92
std::vector< SignatureDataItem > UsedParams
Definition: SimulatorSignature.hpp:145
std::vector< SignatureTypedSpatialDataItem > ProducedVars
Definition: SimulatorSignature.hpp:149
std::vector< SignatureUnitsClassItem > UpdatedUnitsClass
Definition: SimulatorSignature.hpp:230
void clear()
Definition: SimulatorSignature.hpp:176
std::vector< SignatureTypedSpatialDataItem > RequiredVars
Definition: SimulatorSignature.hpp:153
Generated by
1.8.17