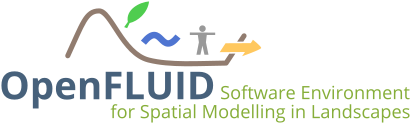 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
40 #ifndef __OPENFLUID_CORE_STRINGVALUE_HPP__
41 #define __OPENFLUID_CORE_STRINGVALUE_HPP__
82 static bool convertStringToDouble(
const std::string& Str,
double& Dbl);
84 static std::vector<std::string>
splitString(
const std::string& StrToSplit,
85 const std::string& Separators,
86 bool ReturnsEmpty =
false);
88 std::vector<std::string> split(
const std::string& Separators,
89 bool ReturnsEmpty =
false)
const;
152 operator std::string()
const
167 bool convert(
Value& Val)
const override;
173 inline std::string
get()
const
191 inline const std::string&
data()
const
200 inline void set(
const std::string& Val)
210 void writeToStream(std::ostream& OutStm)
const override;
214 OutStm <<
"\"" ; writeToStream(OutStm); OutStm <<
"\"" ;
223 return m_Value.size();
232 return m_Value.size();
242 unsigned int replaceAll(
const std::string& FindStr,
const std::string& ReplaceStr);
256 bool toDouble(
double& Val)
const;
270 bool toBoolean(
bool& Val)
const;
284 bool toInteger(
int& Val)
const;
291 bool toInteger(
long& Val)
const;
327 bool toMatrixValue(
const unsigned int& RowLength,
MatrixValue& Val)
const;
334 bool toMapValue(
MapValue& Val)
const;
Definition: VectorValue.hpp:84
Definition: MatrixValue.hpp:84
void set(const std::string &Val)
Definition: StringValue.hpp:200
#define OPENFLUID_API
Definition: dllexport.hpp:86
Type getType() const override
Definition: StringValue.hpp:157
unsigned long size() const
Definition: StringValue.hpp:230
StringValue(const StringValue &Val)
Definition: StringValue.hpp:103
StringValue(const std::string &Val)
Definition: StringValue.hpp:118
std::string get() const
Definition: StringValue.hpp:173
Definition: MapValue.hpp:92
unsigned long getSize() const
Definition: StringValue.hpp:221
void clear()
Definition: StringValue.hpp:205
Type
Definition: Value.hpp:66
Value * clone() const override
Definition: StringValue.hpp:162
Definition: IntegerValue.hpp:79
void writeQuotedToStream(std::ostream &OutStm) const override
Definition: StringValue.hpp:212
std::vector< std::string > OPENFLUID_API splitString(const std::string &StrToSplit, const std::string &Separators, bool ReturnsEmpty=false)
StringValue()
Definition: StringValue.hpp:97
@ STRING
Definition: Value.hpp:66
Definition: DoubleValue.hpp:80
Definition: NullValue.hpp:65
Definition: ApplicationException.hpp:47
StringValue(const char *Val)
Definition: StringValue.hpp:110
std::string & data()
Definition: StringValue.hpp:182
const std::string & data() const
Definition: StringValue.hpp:191
Definition: BooleanValue.hpp:80
Definition: TreeValue.hpp:65
Definition: StringValue.hpp:76
Definition: SimpleValue.hpp:51
Generated by
1.8.17