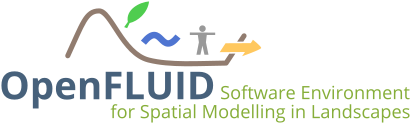 |
Manual for OpenFLUID 2.1.11
|
Go to the documentation of this file.
40 #ifndef __OPENFLUID_CORE_VALUE_HPP__
41 #define __OPENFLUID_CORE_VALUE_HPP__
66 enum Type { NONE, BOOLEAN, INTEGER, DOUBLE, STRING,
VECTOR, MATRIX, MAP, TREE, NULLL };
90 virtual ~
Value() =
default;
92 virtual Type getType()
const = 0;
104 virtual bool isSimple()
const = 0;
106 virtual bool isCompound()
const = 0;
108 virtual void writeToStream(std::ostream& OutStm)
const = 0;
110 virtual void writeQuotedToStream(std::ostream& OutStm)
const = 0;
319 std::string toString()
const;
327 static bool getValueTypeFromString(
const std::string& ValueTypeString,
Value::Type& ValueType);
334 static std::string getStringFromValueType(
const Value::Type ValueType);
Definition: VectorValue.hpp:84
Definition: MatrixValue.hpp:84
virtual bool convert(Value &) const
Definition: Value.hpp:99
@ DOUBLE
Definition: Value.hpp:66
#define OPENFLUID_API
Definition: dllexport.hpp:86
bool isMatrixValue() const
Definition: Value.hpp:253
@ MAP
Definition: Value.hpp:66
bool isStringValue() const
Definition: Value.hpp:187
Definition: MapValue.hpp:92
bool isMapValue() const
Definition: Value.hpp:275
@ TREE
Definition: Value.hpp:66
Type
Definition: Value.hpp:66
virtual Value & operator=(Value &&)
Definition: Value.hpp:85
bool isVectorValue() const
Definition: Value.hpp:231
Definition: IntegerValue.hpp:79
@ STRING
Definition: Value.hpp:66
Definition: DoubleValue.hpp:80
bool isNullValue() const
Definition: Value.hpp:209
virtual void writeToStream(std::ostream &OutStm) const =0
Definition: NullValue.hpp:65
bool isTreeValue() const
Definition: Value.hpp:297
Definition: ApplicationException.hpp:47
virtual Value * clone() const
Definition: Value.hpp:94
@ VECTOR
Definition: Value.hpp:66
@ INTEGER
Definition: Value.hpp:66
bool isIntegerValue() const
Definition: Value.hpp:143
@ NULLL
Definition: Value.hpp:66
Definition: FrameworkException.hpp:50
Definition: BooleanValue.hpp:80
Definition: TreeValue.hpp:65
virtual Value & operator=(const Value &)
Definition: Value.hpp:77
@ MATRIX
Definition: Value.hpp:66
friend std::ostream & operator<<(std::ostream &OutStm, const Value &Val)
Definition: Value.hpp:112
Definition: StringValue.hpp:76
bool isDoubleValue() const
Definition: Value.hpp:121
@ BOOLEAN
Definition: Value.hpp:66
bool isBooleanValue() const
Definition: Value.hpp:165
Generated by
1.8.17